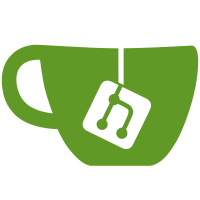
On x86 and AArch, SIMD instructions encode all of the scheduling information in the instruction itself. For example, VADD.I16 q0, q1, q2 is a neon instruction that operates on 16-bit integer elements stored in 128-bit Q registers, which leads to eight 16-bit lanes in parallel. This kind of information impacts how the instruction takes to execute and what dependencies this may cause. On RISCV however, the data that impacts scheduling is encoded in CSR registers such as vtype or vl, in addition with the instruction itself. But MCA does not track or use the data in these registers. This patch fixes this problem by introducing Instruments into MCA. * Replace `CodeRegions` with `AnalysisRegions` * Add `Instrument` and `InstrumentManager` * Add `InstrumentRegions` * Add RISCV Instrument and `InstrumentManager` * Parse `Instruments` in driver * Use instruments to override schedule class * RISCV use lmul instrument to override schedule class * Fix unit tests to pass empty instruments * Add -ignore-im clopt to disable this change A prior version of this patch was commited in5e82ee5373
.2323a4ee61
reverted that change because the unit test files caused build errors. The change with fixes were committed inb88b8307bf
but reverted once againe8e92c8313
due to more build errors. This commit adds the prior changes and fixes the build error. Differential Revision: https://reviews.llvm.org/D137440
58 lines
1.9 KiB
C++
58 lines
1.9 KiB
C++
//===--------------------- CustomBehaviour.cpp ------------------*- C++ -*-===//
|
|
//
|
|
// Part of the LLVM Project, under the Apache License v2.0 with LLVM Exceptions.
|
|
// See https://llvm.org/LICENSE.txt for license information.
|
|
// SPDX-License-Identifier: Apache-2.0 WITH LLVM-exception
|
|
//
|
|
//===----------------------------------------------------------------------===//
|
|
/// \file
|
|
///
|
|
/// This file implements methods from the CustomBehaviour interface.
|
|
///
|
|
//===----------------------------------------------------------------------===//
|
|
|
|
#include "llvm/MCA/CustomBehaviour.h"
|
|
|
|
namespace llvm {
|
|
namespace mca {
|
|
|
|
CustomBehaviour::~CustomBehaviour() = default;
|
|
|
|
unsigned CustomBehaviour::checkCustomHazard(ArrayRef<InstRef> IssuedInst,
|
|
const InstRef &IR) {
|
|
// 0 signifies that there are no hazards that need to be waited on
|
|
return 0;
|
|
}
|
|
|
|
std::vector<std::unique_ptr<View>>
|
|
CustomBehaviour::getStartViews(llvm::MCInstPrinter &IP,
|
|
llvm::ArrayRef<llvm::MCInst> Insts) {
|
|
return std::vector<std::unique_ptr<View>>();
|
|
}
|
|
|
|
std::vector<std::unique_ptr<View>>
|
|
CustomBehaviour::getPostInstrInfoViews(llvm::MCInstPrinter &IP,
|
|
llvm::ArrayRef<llvm::MCInst> Insts) {
|
|
return std::vector<std::unique_ptr<View>>();
|
|
}
|
|
|
|
std::vector<std::unique_ptr<View>>
|
|
CustomBehaviour::getEndViews(llvm::MCInstPrinter &IP,
|
|
llvm::ArrayRef<llvm::MCInst> Insts) {
|
|
return std::vector<std::unique_ptr<View>>();
|
|
}
|
|
|
|
SharedInstrument InstrumentManager::createInstrument(llvm::StringRef Desc,
|
|
llvm::StringRef Data) {
|
|
return std::make_shared<Instrument>(Desc, Data);
|
|
}
|
|
|
|
unsigned InstrumentManager::getSchedClassID(
|
|
const MCInstrInfo &MCII, const MCInst &MCI,
|
|
const llvm::SmallVector<SharedInstrument> &IVec) const {
|
|
return MCII.get(MCI.getOpcode()).getSchedClass();
|
|
}
|
|
|
|
} // namespace mca
|
|
} // namespace llvm
|