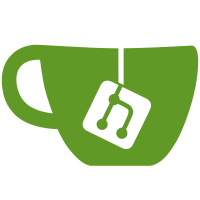
There are 12bit offset fields in the ld.[b/h/w/d] and st.[b/h/w/d]. When the constant address is less than 12 bits, the address calculation is incorporated into the offset field of the instruction. Differential Revision: https://reviews.llvm.org/D143470
66 lines
2.2 KiB
C++
66 lines
2.2 KiB
C++
//=- LoongArchISelDAGToDAG.h - A dag to dag inst selector for LoongArch ---===//
|
|
//
|
|
// Part of the LLVM Project, under the Apache License v2.0 with LLVM Exceptions.
|
|
// See https://llvm.org/LICENSE.txt for license information.
|
|
// SPDX-License-Identifier: Apache-2.0 WITH LLVM-exception
|
|
//
|
|
//===----------------------------------------------------------------------===//
|
|
//
|
|
// This file defines an instruction selector for the LoongArch target.
|
|
//
|
|
//===----------------------------------------------------------------------===//
|
|
|
|
#ifndef LLVM_LIB_TARGET_LOONGARCH_LOONGARCHISELDAGTODAG_H
|
|
#define LLVM_LIB_TARGET_LOONGARCH_LOONGARCHISELDAGTODAG_H
|
|
|
|
#include "LoongArch.h"
|
|
#include "LoongArchTargetMachine.h"
|
|
#include "llvm/CodeGen/SelectionDAGISel.h"
|
|
|
|
// LoongArch-specific code to select LoongArch machine instructions for
|
|
// SelectionDAG operations.
|
|
namespace llvm {
|
|
class LoongArchDAGToDAGISel : public SelectionDAGISel {
|
|
const LoongArchSubtarget *Subtarget = nullptr;
|
|
|
|
public:
|
|
static char ID;
|
|
|
|
LoongArchDAGToDAGISel() = delete;
|
|
|
|
explicit LoongArchDAGToDAGISel(LoongArchTargetMachine &TM)
|
|
: SelectionDAGISel(ID, TM) {}
|
|
|
|
bool runOnMachineFunction(MachineFunction &MF) override {
|
|
Subtarget = &MF.getSubtarget<LoongArchSubtarget>();
|
|
return SelectionDAGISel::runOnMachineFunction(MF);
|
|
}
|
|
|
|
void Select(SDNode *Node) override;
|
|
|
|
bool SelectInlineAsmMemoryOperand(const SDValue &Op, unsigned ConstraintID,
|
|
std::vector<SDValue> &OutOps) override;
|
|
|
|
bool SelectBaseAddr(SDValue Addr, SDValue &Base);
|
|
bool SelectAddrConstant(SDValue Addr, SDValue &Base, SDValue &Offset);
|
|
bool selectNonFIBaseAddr(SDValue Addr, SDValue &Base);
|
|
|
|
bool selectShiftMask(SDValue N, unsigned ShiftWidth, SDValue &ShAmt);
|
|
bool selectShiftMaskGRLen(SDValue N, SDValue &ShAmt) {
|
|
return selectShiftMask(N, Subtarget->getGRLen(), ShAmt);
|
|
}
|
|
bool selectShiftMask32(SDValue N, SDValue &ShAmt) {
|
|
return selectShiftMask(N, 32, ShAmt);
|
|
}
|
|
|
|
bool selectSExti32(SDValue N, SDValue &Val);
|
|
bool selectZExti32(SDValue N, SDValue &Val);
|
|
|
|
// Include the pieces autogenerated from the target description.
|
|
#include "LoongArchGenDAGISel.inc"
|
|
};
|
|
|
|
} // end namespace llvm
|
|
|
|
#endif // LLVM_LIB_TARGET_LOONGARCH_LOONGARCHISELDAGTODAG_H
|