mirror of
https://github.com/go-sylixos/elvish.git
synced 2024-12-05 03:17:50 +08:00
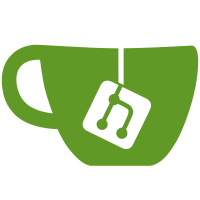
This feature supersedes the CompileWithGlobal method, and simplifies the only use of that method in pkg/edit, where the default binding is evaluated using the edit: namespace as the global Ns.
93 lines
1.9 KiB
Go
93 lines
1.9 KiB
Go
package eval
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/elves/elvish/pkg/parse"
|
|
)
|
|
|
|
func BenchmarkEval_Empty(b *testing.B) {
|
|
benchmarkEval(b, "")
|
|
}
|
|
|
|
func BenchmarkEval_NopCommand(b *testing.B) {
|
|
benchmarkEval(b, "nop")
|
|
}
|
|
|
|
func BenchmarkEval_PutCommand(b *testing.B) {
|
|
benchmarkEval(b, "put x")
|
|
}
|
|
|
|
func BenchmarkEval_ForLoop100WithEmptyBody(b *testing.B) {
|
|
benchmarkEval(b, "for x [(range 100)] { }")
|
|
}
|
|
|
|
func BenchmarkEval_EachLoop100WithEmptyBody(b *testing.B) {
|
|
benchmarkEval(b, "range 100 | each [x]{ }")
|
|
}
|
|
|
|
func BenchmarkEval_LocalVariableAccess(b *testing.B) {
|
|
benchmarkEval(b, "x = val; nop $x")
|
|
}
|
|
|
|
func BenchmarkEval_UpVariableAccess(b *testing.B) {
|
|
benchmarkEval(b, "x = val; { nop $x }")
|
|
}
|
|
|
|
func benchmarkEval(b *testing.B, code string) {
|
|
ev := NewEvaler()
|
|
src := parse.Source{Name: "[benchmark]", Code: code}
|
|
|
|
tree, err := parse.Parse(src)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
op, err := ev.compile(tree, ev.Global, nil)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
b.ResetTimer()
|
|
|
|
for i := 0; i < b.N; i++ {
|
|
ev.execOp(op, EvalCfg{Global: ev.Global})
|
|
}
|
|
}
|
|
|
|
func BenchmarkOutputCapture_Overhead(b *testing.B) {
|
|
benchmarkOutputCapture(b.N, func(fm *Frame) {})
|
|
}
|
|
|
|
func BenchmarkOutputCapture_Values(b *testing.B) {
|
|
benchmarkOutputCapture(b.N, func(fm *Frame) {
|
|
fm.OutputChan() <- "test"
|
|
})
|
|
}
|
|
|
|
func BenchmarkOutputCapture_Bytes(b *testing.B) {
|
|
bytesToWrite := []byte("test")
|
|
benchmarkOutputCapture(b.N, func(fm *Frame) {
|
|
fm.OutputFile().Write(bytesToWrite)
|
|
})
|
|
}
|
|
|
|
func BenchmarkOutputCapture_Mixed(b *testing.B) {
|
|
bytesToWrite := []byte("test")
|
|
benchmarkOutputCapture(b.N, func(fm *Frame) {
|
|
fm.OutputChan() <- false
|
|
fm.OutputFile().Write(bytesToWrite)
|
|
})
|
|
}
|
|
|
|
func benchmarkOutputCapture(n int, f func(*Frame)) {
|
|
ev := NewEvaler()
|
|
defer ev.Close()
|
|
fm := NewTopFrame(ev, parse.Source{Name: "[benchmark]"}, []*Port{{}, {}, {}})
|
|
for i := 0; i < n; i++ {
|
|
fm.CaptureOutput(func(fm *Frame) error {
|
|
f(fm)
|
|
return nil
|
|
})
|
|
}
|
|
}
|