mirror of
https://github.com/go-sylixos/elvish.git
synced 2024-12-04 02:37:50 +08:00
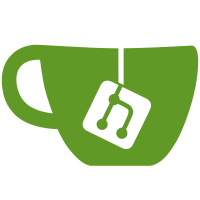
The elvdocs still use the old format (#elvdoc:fn or #elvdoc:var) for now, but will be changed to "fn" and "var" forms soon. Also remove the accidentally committed cmd/mvelvdoc. It has been used to perform the conversion automatically but is not supposed to be committed.
33 lines
591 B
Go
33 lines
591 B
Go
package eval
|
|
|
|
import (
|
|
"errors"
|
|
"os"
|
|
)
|
|
|
|
// ErrNonExistentEnvVar is raised by the get-env command when the environment
|
|
// variable does not exist.
|
|
var ErrNonExistentEnvVar = errors.New("non-existent environment variable")
|
|
|
|
func init() {
|
|
addBuiltinFns(map[string]any{
|
|
"has-env": hasEnv,
|
|
"get-env": getEnv,
|
|
"set-env": os.Setenv,
|
|
"unset-env": os.Unsetenv,
|
|
})
|
|
}
|
|
|
|
func hasEnv(key string) bool {
|
|
_, ok := os.LookupEnv(key)
|
|
return ok
|
|
}
|
|
|
|
func getEnv(key string) (string, error) {
|
|
value, ok := os.LookupEnv(key)
|
|
if !ok {
|
|
return "", ErrNonExistentEnvVar
|
|
}
|
|
return value, nil
|
|
}
|